How to Get LinkedIn Profile Data Using API (No OAuth Required)
Learn how to access LinkedIn profile data programmatically without the complexity of OAuth authentication. This guide covers practical methods, tools, and best practices.
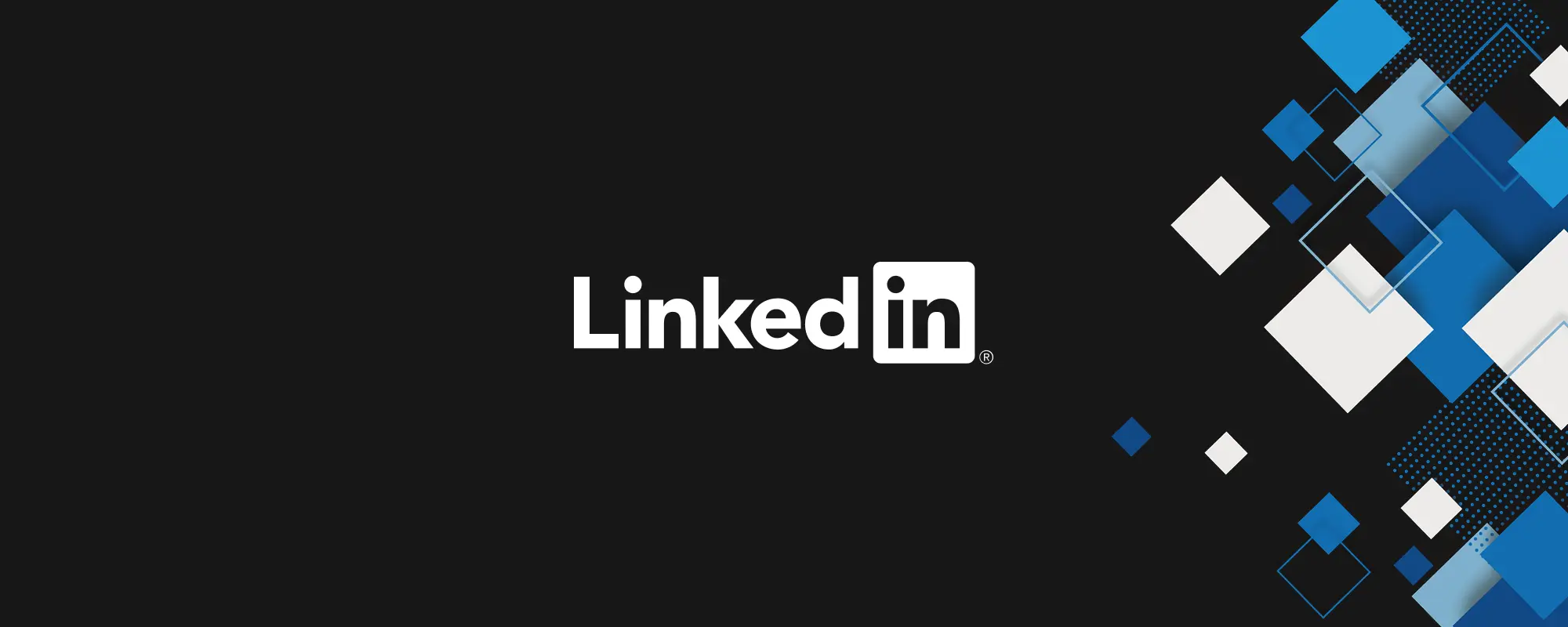
How to Get LinkedIn Profile Data Using API (No OAuth Required)
In this comprehensive guide, you'll discover:
- Why accessing LinkedIn data is valuable for businesses and developers
- Methods to obtain LinkedIn profile data without OAuth authentication
- Step-by-step implementation with code examples
- Best practices and ethical considerations
Why Access to LinkedIn API?
LinkedIn is the world's largest professional network with over 900 million members across 200 countries. This massive pool of professional data is incredibly valuable for:
- Recruiters and HR professionals: Finding qualified candidates and verifying employment history
- Sales teams: Identifying prospects and understanding their professional background
- Marketers: Building targeted campaigns based on job titles, industries, and company sizes
- Researchers: Analyzing professional networks and career trajectories
While LinkedIn offers an official API, it's restricted in scope and requires OAuth authentication, which can be complex to implement and requires approval through LinkedIn's developer program. Fortunately, there are alternative approaches for accessing LinkedIn profile data without OAuth.
How to Access LinkedIn API?
Before diving into non-OAuth methods, it's important to understand your options:
1. Official LinkedIn API (with OAuth)
LinkedIn's official Developer Platform offers APIs for:
- Sign In with LinkedIn
- Share on LinkedIn
- Marketing Developer Platform
- Compliance APIs
These require OAuth 2.0 authentication and application approval, with strict usage policies.
2. Third-Party LinkedIn Data Providers
Several services specialize in providing LinkedIn data through simplified APIs:
- People Data Labs: Offers enrichment APIs with LinkedIn profile information
- Proxycurl: Provides LinkedIn-specific APIs without OAuth
- Lusha: Focuses on contact information from LinkedIn profiles
- Apollo.io: Combines LinkedIn and other business data sources
- RapidAPI: Marketplace hosting multiple LinkedIn data APIs including Fresh LinkedIn Scraper API
Many of these services offer free tiers or trial credits, making them accessible for testing before committing to a paid plan.
3. Web Scraping Approaches
While technically possible, direct scraping of LinkedIn:
- Violates LinkedIn's Terms of Service
- Faces sophisticated anti-scraping measures
- Risks account blocking and potential legal issues
We'll focus on the second option—using third-party APIs that handle the complexities for you.
Step by Step Guide
Let's implement solutions using third-party API services that don't require OAuth authentication.
Using the Fresh LinkedIn Scraper API on RapidAPI
RapidAPI hosts numerous LinkedIn data services, including the Fresh LinkedIn Scraper API, which offers simple endpoints for accessing LinkedIn profile and company data. Let's see how to use it:
Step 1: Set Up Your RapidAPI Account
- Visit RapidAPI and create an account
- Subscribe to the Fresh LinkedIn Scraper API
- Obtain your API key from the RapidAPI dashboard
Step 2: Using the API with Python
Here's how to fetch LinkedIn profile data using Python:
import requests
url = "https://fresh-linkedin-scraper-api.p.rapidapi.com/api/v1/user/profile"
querystring = {"username":"williamhgates"}
headers = {
"x-rapidapi-key": "<Your api key>",
"x-rapidapi-host": "fresh-linkedin-scraper-api.p.rapidapi.com"
}
response = requests.get(url, headers=headers, params=querystring)
print(response.json())
This will return detailed profile information in JSON format. Here's an example response:
{
"success": true,
"message": "success",
"process_time": 468,
"cost": 1,
"data": {
"id": "251749025",
"urn": "ACoAAA8BYqEBCGLg_vT_ca6mMEqkpp9nVffJ3hc",
"public_identifier": "williamhgates",
"first_name": "Bill",
"last_name": "Gates",
"full_name": "Bill Gates",
"headline": "Chair, Gates Foundation and Founder, Breakthrough Energy",
"is_premium": true,
"is_open_to_work": false,
"is_hiring": false,
"is_memorialized": false,
"is_influencer": true,
"is_top_voice": true,
"is_creator": true,
"birth": {
"day": null,
"month": null,
"year": null
},
"pronoun": null,
"created": 1367521780840,
"created_date": "2013-05-02T19:09:40.840Z",
"location": {
"country": "United States",
"country_code": "US",
"city": "Seattle, Washington, United States",
"postal_code": null
},
"avatar": [
{
"width": 200,
"height": 200,
"url": "https://media.licdn.com/dms/image/v2/D5603AQF-RYZP55jmXA/profile-displayphoto-shrink_200_200/B56ZRi8g.aGsAY-/0/1736826818802?e=1749081600&v=beta&t=8hZGHekNOCdOgVucnR22cbwafY_kXLCmIDVl3DGkfSc",
"expires_at": 1749081600000
},
{
"width": 400,
"height": 400,
"url": "https://media.licdn.com/dms/image/v2/D5603AQF-RYZP55jmXA/profile-displayphoto-shrink_400_400/B56ZRi8g.aGsAg-/0/1736826818802?e=1749081600&v=beta&t=COIAnqFqPM_6v8kTUnIdUV0ymqE-iv8ehHl_Kr0h5SQ",
"expires_at": 1749081600000
},
{
"width": 100,
"height": 100,
"url": "https://media.licdn.com/dms/image/v2/D5603AQF-RYZP55jmXA/profile-displayphoto-shrink_100_100/B56ZRi8g.aGsAU-/0/1736826818802?e=1749081600&v=beta&t=hd4wzNKgmLsnVnM3UrvKucxHcLYV2m7Az4aB-AFHrq8",
"expires_at": 1749081600000
},
{
"width": 800,
"height": 800,
"url": "https://media.licdn.com/dms/image/v2/D5603AQF-RYZP55jmXA/profile-displayphoto-shrink_800_800/B56ZRi8g.aGsAc-/0/1736826818808?e=1749081600&v=beta&t=NbWZseAYCZLDFblO75TLDt0LEGF5kjLB3mOkve_XNrg",
"expires_at": 1749081600000
}
],
"cover": [
{
"width": 800,
"height": 200,
"url": "https://media.licdn.com/dms/image/v2/D5616AQEyKmz7jPZung/profile-displaybackgroundimage-shrink_200_800/profile-displaybackgroundimage-shrink_200_800/0/1737141827999?e=1749081600&v=beta&t=6vV54NhapnL2PqnzQH6mgBJzcQmzwY-ekqwcu1zkORQ",
"expires_at": 1749081600000
},
{
"width": 1400,
"height": 350,
"url": "https://media.licdn.com/dms/image/v2/D5616AQEyKmz7jPZung/profile-displaybackgroundimage-shrink_350_1400/profile-displaybackgroundimage-shrink_350_1400/0/1737141827999?e=1749081600&v=beta&t=OkbwMcdTgO3hoCPxhkp2cA18p6jo7y8r5EcvyAlIZLQ",
"expires_at": 1749081600000
}
],
"associated_hashtag": [
"#books",
"#healthcare",
"#innovation",
"#climatechange",
"#sustainability"
],
"website": {},
"supported_locales": [
{
"country": "US",
"language": "en"
}
],
"primary_locale": {
"country": "US",
"language": "en"
}
}
}
For company data, you can use the company endpoint:
import requests
url = "https://fresh-linkedin-scraper-api.p.rapidapi.com/api/v1/company/profile"
querystring = {"company":"rapidapi"}
headers = {
"x-rapidapi-key": "<Your api key>",
"x-rapidapi-host": "fresh-linkedin-scraper-api.p.rapidapi.com"
}
response = requests.get(url, headers=headers, params=querystring)
print(response.json())
Example company data response:
{
"success": true,
"message": "success",
"process_time": 888,
"cost": 1,
"data": {
"id": "10649600",
"name": "Rapid",
"universal_name": "rapidapi",
"description": "RapidAPI is the world's largest API Hub where millions of developers find and connect to tens of thousands of public APIs. \n\nWe're a team of developers, building for developers, based in San Francisco, Tel Aviv, Tallinn, Berlin, and remote locations around the world.",
"linkedin_url": "https://www.linkedin.com/company/rapidapi/",
"website_url": "https://RapidAPI.com/",
"phone": null,
"active": true,
"archived": false,
"follower_count": 24383,
"employee_count": 351,
"employee_count_range": {
"start": 51,
"end": 200
},
"verification": {
"verified": true,
"last_modified_at": "2023-09-12T18:18:31.454Z"
},
"founded_on": {
"day": null,
"month": null,
"year": 2015
},
"headquarter": {
"country": "US",
"city": "San Francisco",
"geographic_area": "California",
"line1": "2 Shaw Alley",
"line2": "Floor #4",
"postal_code": "94105"
},
"locations": [
{
"name": "Berlin",
"address": {
"country": "DE",
"city": "Berlin",
"geographic_area": null
},
"lat_long": {
"latitude": 52.560253,
"longitude": 13.296672
}
},
{
"name": "San Francisco",
"address": {
"country": "US",
"city": "San Francisco",
"geographic_area": "California",
"line1": "2 Shaw Alley",
"line2": "Floor #4",
"postal_code": "94105"
},
"lat_long": {
"latitude": 37.39271,
"longitude": -122.042
}
},
{
"name": "Tallinn",
"address": {
"country": "EE",
"city": "Tallinn",
"geographic_area": null
},
"lat_long": {
"latitude": 59.437214,
"longitude": 24.745369
}
},
{
"name": "Tel Aviv",
"address": {
"country": "IL",
"city": "Tel Aviv",
"geographic_area": null,
"line1": "Menachem Begin Blv. 148"
},
"lat_long": {
"latitude": 30.812426,
"longitude": 34.859478
}
}
],
"logo": [
{
"width": 200,
"height": 200,
"url": "https://media.licdn.com/dms/image/v2/D560BAQHnqRt58Ae7ig/company-logo_200_200/company-logo_200_200/0/1731507876313/rapidapi_logo?e=1749081600&v=beta&t=YGqYWDGZrRukb8R_vawcfMSySySBX_fdHh5renY2mD4",
"expires_at": 1749081600000
},
{
"width": 100,
"height": 100,
"url": "https://media.licdn.com/dms/image/v2/D560BAQHnqRt58Ae7ig/company-logo_100_100/company-logo_100_100/0/1731507876313/rapidapi_logo?e=1749081600&v=beta&t=Z8xtb2oMEJc2UiCvBah0uiefkDuPqEnHsaQYabzS82o",
"expires_at": 1749081600000
},
{
"width": 400,
"height": 400,
"url": "https://media.licdn.com/dms/image/v2/D560BAQHnqRt58Ae7ig/company-logo_400_400/company-logo_400_400/0/1731507876313/rapidapi_logo?e=1749081600&v=beta&t=ohr6N4R_CuHgPMNg6Wy3fRndPWOzW2mcYmDyQ3pWF38",
"expires_at": 1749081600000
}
],
"cover": [
{
"width": 1920,
"height": 1080,
"url": "https://media.licdn.com/dms/image/v2/D561BAQGyzYbC2bAI5g/company-background_10000/company-background_10000/0/1731507926382/rapidapi_cover?e=1744012800&v=beta&t=YJvorvFsD2dz02nTiDcag1gwRxsqJzklsL_hADalBZ8",
"expires_at": 1744012800000
},
{
"width": 400,
"height": 225,
"url": "https://media.licdn.com/dms/image/v2/D561BAQGyzYbC2bAI5g/company-background_400/company-background_400/0/1731507926382/rapidapi_cover?e=1744012800&v=beta&t=T57DJ16XStQysNeGBy1rdJShoHETkwLyDPJM45jtk1I",
"expires_at": 1744012800000
},
{
"width": 200,
"height": 112,
"url": "https://media.licdn.com/dms/image/v2/D561BAQGyzYbC2bAI5g/company-background_200/company-background_200/0/1731507926382/rapidapi_cover?e=1744012800&v=beta&t=l07Zn3goeEQXGYIrHKy11pEMMlJIfivuC0rZknD1cJo",
"expires_at": 1744012800000
}
],
"specialities": [
"API Hub",
"API",
"Web",
"Developers",
"API Client",
"REST API",
"GraphQL",
"Kafka",
"OpenAPI",
"Application Programming Interface",
"Web APIs",
"JavaScript",
"React",
"Vue",
"Python",
"Java",
"PHP",
"C",
"C#",
"C++",
"TypeScript",
".NET"
],
"industries": ["Information Technology & Services"],
"hashtags": [
{
"name": "#dev",
"follower_count": 12600
},
{
"name": "#rapidapi",
"follower_count": 36
},
{
"name": "#api",
"follower_count": 14838
}
],
"funding_info": {
"number_of_funding_rounds": 7,
"organization_url": "https://www.crunchbase.com/organization/rapidapi?utm_source=linkedin&utm_medium=referral&utm_campaign=linkedin_companies&utm_content=profile_cta",
"funding_rounds_url": "https://www.crunchbase.com/organization/rapidapi/funding_rounds/funding_rounds_list?utm_source=linkedin&utm_medium=referral&utm_campaign=linkedin_companies&utm_content=all_fundings",
"updated_at": "2022-11-23T02:57:50.000Z",
"last_funding_round": {
"type": "Series D",
"money_raised": {
"amount": "150000000",
"currency": "USD"
},
"url": "https://www.crunchbase.com/funding_round/rapidapi-series-d--8c1d29e4?utm_source=linkedin&utm_medium=referral&utm_campaign=linkedin_companies&utm_content=last_funding",
"investors_url": "https://www.crunchbase.com/funding_round/rapidapi-series-d--8c1d29e4?utm_source=linkedin&utm_medium=referral&utm_campaign=linkedin_companies&utm_content=all_investors",
"number_of_other_investors": 6,
"announced_on": {
"day": 23,
"month": 3,
"year": 2022
}
}
}
}
}
Step 4: Available Endpoints
The Fresh LinkedIn Scraper API offers a comprehensive set of endpoints organized by category:
Job Endpoints
- Search Jobs: Search for jobs by keywords, titles, or descriptions
- Job Detail: Get detailed information about a specific job by ID
Post Endpoints
- Post Detail: Retrieve complete information about a LinkedIn post
- Post Comments: Get comments on a specific LinkedIn post
- Post Reactions: View reaction data (likes, etc.) for a post
- Post Reposts: See who has reshared a particular post
User Profile Data Endpoints
- User Profile: Get core profile information (name, headline, about, etc.)
- User Contact: Retrieve available contact information
- User Posts: Get a list of posts published by the user
- User Comments: View comments made by the user
- User Videos: Get videos shared by the user
- User Images: Retrieve images shared by the user
- User Reactions: See posts the user has reacted to
- User Documents: Get documents shared by the user
- User Recommendations: View recommendations received and given
- Save User Profile to PDF: Generate a PDF version of a user's profile
Group Endpoints
- Group Info: Get information about a LinkedIn group
- Group Posts: Retrieve posts from a specific group
Search Endpoints
- Search Posts: Find posts by keywords and other criteria
- Search Locations: Search for locations on LinkedIn
Ads Endpoints
- Search Ads: Find LinkedIn ads by various criteria
- Ad Detail: Get detailed information about a specific ad
Company Endpoints
- Company Profile: Retrieve comprehensive company information
- Company Posts: Get posts published by a company
User Additional Data Endpoints
- User Skills: Get a list of skills listed on a user's profile
- User Education: View educational background details
- User Licenses and Certifications: Get professional certifications
- User Publications: Retrieve published works listed on profile
- User Honors: Get awards and recognition information
- User Experiences: View work experience history
- User Volunteers: Get volunteer experience information
- User Follower and Connection: View follower and connection data
For detailed documentation on request parameters and response formats, refer to the RapidAPI interface.
This enhanced version:
- Creates a reusable LinkedIn API class
- Handles both profile and company data
- Extracts the most relevant information
- Processes multiple profiles
- Saves results to a JSON file
Final Thoughts
While the official LinkedIn API with OAuth offers the most compliant way to access LinkedIn data, third-party APIs provide a practical alternative when OAuth implementation is too complex or your use case doesn't fit LinkedIn's partner programs.
Remember these key points:
-
Legal and ethical considerations: Always review the terms of service for any API you use. Most third-party providers comply with data privacy regulations and LinkedIn's terms by using legitimate methods of data collection.
-
Cost efficiency: These services are not free, but they typically offer pay-as-you-go models that may be more cost-effective than building and maintaining your own data collection infrastructure.
-
Data freshness: The quality of data varies by provider. Some offer real-time scraping while others maintain databases that may be updated less frequently.
-
Rate limits and quotas: Be mindful of your API usage to avoid unexpected costs or service limitations.
-
Privacy compliance: Ensure your data usage complies with regulations like GDPR, CCPA, and other applicable laws.
By leveraging these non-OAuth LinkedIn data solutions, you can access valuable professional data while focusing your development efforts on creating value with that data, rather than on the complex challenges of data acquisition.
Have you integrated LinkedIn data into your applications? What approaches have you found most effective? Share your experiences and questions in the comments below!