LinkedIn Public Profile Scraping with Node.js
Learn how to scrape LinkedIn public profiles using Node.js and the Fresh LinkedIn Scraper API through RapidAPI. Get step-by-step instructions with code examples.
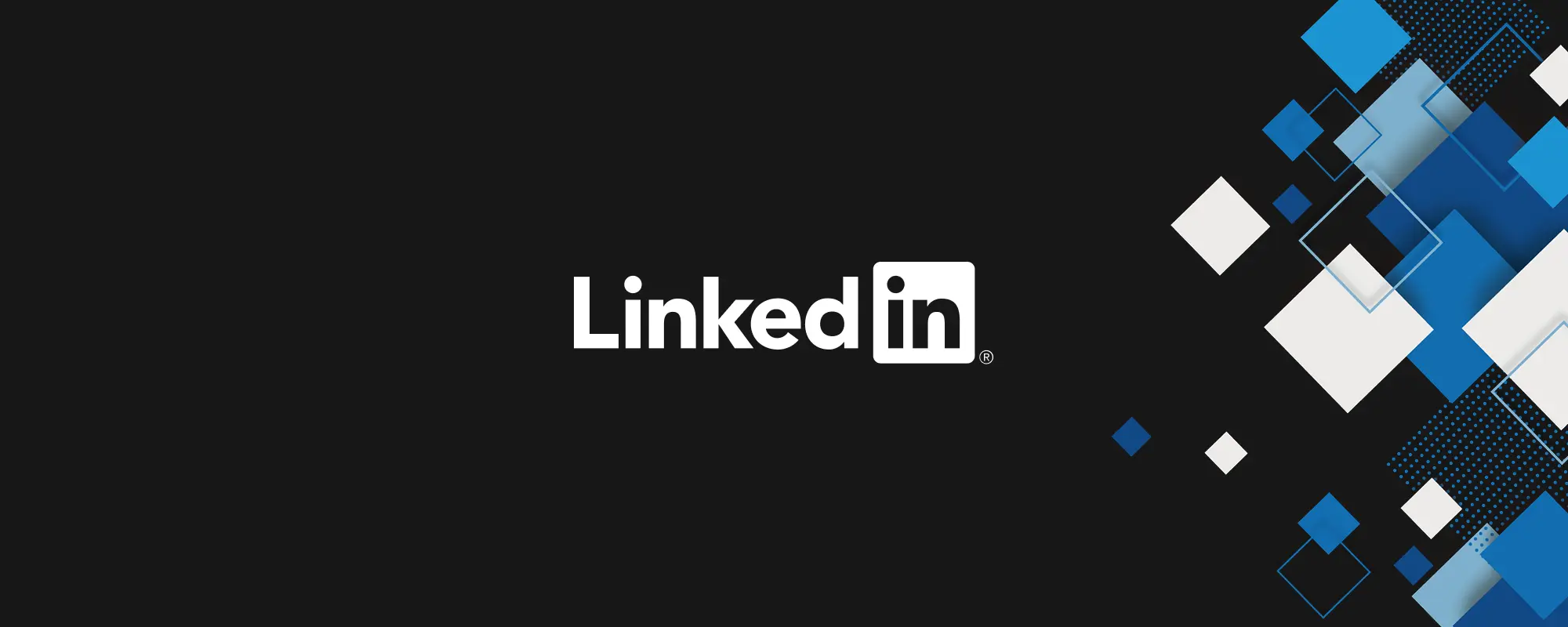
LinkedIn Public Profile Scraping with Node.js
In this comprehensive guide, you'll learn how to scrape LinkedIn public profiles using Node.js and the Fresh LinkedIn Scraper API. By the end of this tutorial, you'll be able to programmatically access LinkedIn profile data without the complexity of OAuth authentication.
Table of Contents
- Why Access LinkedIn Profile Data?
- Available Methods for LinkedIn Data Access
- Using Fresh LinkedIn Scraper API
- Step-by-Step Implementation with Node.js
- Working with the API Response
- Best Practices and Limitations
- Alternative Approaches
- Conclusion
Why Access LinkedIn Profile Data?
LinkedIn is the world's premier professional network with nearly a billion members globally. Access to LinkedIn profile data can be valuable for numerous business applications:
- Recruitment & HR: Verify candidate work histories and skills
- Sales & Marketing: Generate leads and research prospects
- Business Development: Identify potential partners and collaborators
- Research: Analyze professional networks and industry trends
While LinkedIn offers an official API, its restrictions and OAuth requirements can be challenging for many use cases. This is where alternative solutions like the Fresh LinkedIn Scraper API come in.
Available Methods for LinkedIn Data Access
When it comes to accessing LinkedIn profile data, you have several options:
- LinkedIn's Official API: Requires OAuth 2.0 authorization, has limited scope, and needs application approval
- Third-Party LinkedIn Data APIs: Simplified access without OAuth complications
- Custom Web Scraping: Building your own scraping solution (not recommended due to legal and technical challenges)
For this tutorial, we'll focus on the second approach using the Fresh LinkedIn Scraper API available on RapidAPI.
Using Fresh LinkedIn Scraper API
The Fresh LinkedIn Scraper API offers a straightforward way to access LinkedIn profile data without dealing with complex authentication flows or scraping challenges. Key benefits include:
- No OAuth authentication required
- Simple REST API interface
- Comprehensive profile data extraction
- Rate limiting protection
- Regular updates to maintain reliability
Step-by-Step Implementation with Node.js
Let's implement a solution to fetch LinkedIn profile data using Node.js and axios.
Step 1: Set Up Your Project
First, create a new Node.js project and install the required dependencies:
mkdir linkedin-profile-scraper
cd linkedin-profile-scraper
npm init -y
npm install axios
Step 2: Create the Scraper Script
Create a file named scraper.js
and add the following code:
const axios = require("axios");
const options = {
method: "GET",
url: "https://fresh-linkedin-scraper-api.p.rapidapi.com/api/v1/user/profile",
params: {
username: "williamhgates",
},
headers: {
"x-rapidapi-key": "YOUR_API_KEY",
"x-rapidapi-host": "fresh-linkedin-scraper-api.p.rapidapi.com",
},
};
async function fetchData() {
try {
const response = await axios.request(options);
console.log(response.data);
} catch (error) {
console.error(error);
}
}
fetchData();
Note: Replace the API key with your own from RapidAPI.
Step 3: Run the Scraper
Execute the script with Node.js:
node scraper.js
If everything is set up correctly, you should see a JSON response with detailed profile information for Bill Gates.
Working with the API Response
The API returns a rich JSON structure containing various profile details. Here's a breakdown of key information you can access:
// Example of extracting specific profile information
async function fetchAndProcessData() {
try {
const response = await axios.request(options);
const profile = response.data.data;
// Basic profile information
console.log(`Name: ${profile.full_name}`);
console.log(`Headline: ${profile.headline}`);
console.log(`Location: ${profile.location.city}`);
// Work experience
if (profile.experience && profile.experience.length > 0) {
console.log("\nWork Experience:");
profile.experience.forEach((job) => {
console.log(`- ${job.title} at ${job.company}`);
});
}
// Education
if (profile.education && profile.education.length > 0) {
console.log("\nEducation:");
profile.education.forEach((edu) => {
console.log(`- ${edu.degree_name} at ${edu.school}`);
});
}
} catch (error) {
console.error("Error fetching LinkedIn data:", error);
}
}
Sample Response Structure
The API response typically contains the following key sections:
- Basic Information: Name, headline, location, etc.
- Experience: Work history with companies, roles, and dates
- Education: Academic background
- Skills: Listed professional skills
- Certifications: Professional certifications
- Recommendations: Received and given recommendations
- Accomplishments: Publications, patents, courses, etc.
- Interests: Companies, groups, and schools followed
Best Practices and Limitations
When using the LinkedIn Profile Scraper API, keep these best practices in mind:
Rate Limiting
Most API providers implement rate limits. Check your RapidAPI dashboard for your specific limits and monitor your usage to avoid disruptions.
Error Handling
Implement robust error handling to manage API downtime, rate limiting, and network issues:
async function robustFetch() {
try {
const response = await axios.request(options);
return response.data;
} catch (error) {
if (error.response) {
// The request was made and the server responded with a status code
// that falls out of the range of 2xx
console.error(
"Error response:",
error.response.status,
error.response.data
);
} else if (error.request) {
// The request was made but no response was received
console.error("No response received:", error.request);
} else {
// Something happened in setting up the request that triggered an Error
console.error("Request error:", error.message);
}
return null;
}
}
Caching Results
To minimize API calls, consider implementing a caching mechanism:
const NodeCache = require("node-cache");
const profileCache = new NodeCache({ stdTTL: 86400 }); // Cache for 24 hours
async function fetchProfileWithCache(username) {
// Check if profile is in cache
const cachedProfile = profileCache.get(username);
if (cachedProfile) {
console.log("Retrieved from cache");
return cachedProfile;
}
// Fetch from API if not in cache
try {
options.params.username = username;
const response = await axios.request(options);
const profile = response.data;
// Store in cache
profileCache.set(username, profile);
return profile;
} catch (error) {
console.error("Error fetching profile:", error);
return null;
}
}
Alternative Approaches
If the Fresh LinkedIn Scraper API doesn't meet your specific needs, consider these alternatives:
- Proxycurl: Another popular LinkedIn data provider with comprehensive APIs
- People Data Labs: Offers enrichment APIs that include LinkedIn profile information
- Lusha: Specializes in contact information extraction
- Apollo.io: Combines LinkedIn and other business data sources
Each service has different pricing models and data coverage, so evaluate based on your specific requirements.
Conclusion
With the Fresh LinkedIn Scraper API and Node.js, you can easily access public LinkedIn profile data without the complexity of OAuth authentication or the challenges of building your own scraping solution.
This approach allows you to focus on using the data for your applications rather than worrying about the technical challenges of data extraction. Whether you're building a recruitment tool, sales intelligence platform, or research application, this API provides a reliable way to incorporate LinkedIn profile data.
Remember to respect LinkedIn's terms of service and user privacy when working with profile data, and always ensure your use case complies with relevant data protection regulations.
Ready to get started? Sign up for the Fresh LinkedIn Scraper API on RapidAPI and begin integrating LinkedIn profile data into your Node.js applications today.