LinkedIn API Search by Keyword: Find Professionals Programmatically
Learn how to search LinkedIn profiles by keyword using our API without OAuth authentication. Access comprehensive professional data with simple REST calls.
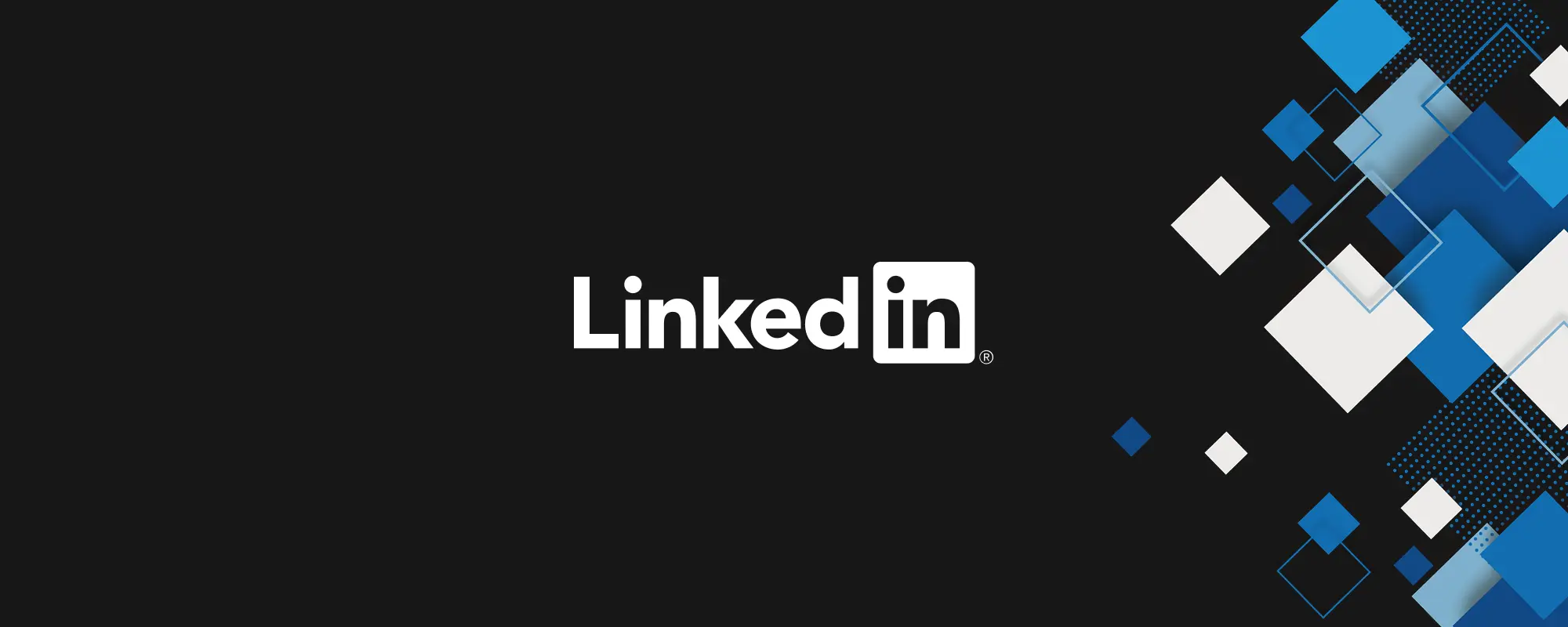
LinkedIn API Search by Keyword: Find Professionals Programmatically
Searching for professionals on LinkedIn programmatically has traditionally required complex OAuth authentication and approval processes. Our Fresh LinkedIn Scraper API on RapidAPI solves this challenge by providing a simple endpoint to search LinkedIn profiles by keyword without authentication barriers.
Table of Contents
- How LinkedIn API Search Works
- Making Your First API Call
- Understanding the Response
- Advanced Search Options
- Use Cases
- Getting Started
- FAQ
How LinkedIn API Search Works
The people search endpoint allows you to programmatically find LinkedIn profiles using keywords, similar to LinkedIn's own search functionality. The API returns structured data about professionals matching your search criteria, including their names, titles, locations, and profile URLs.
Unlike LinkedIn's official API, our solution:
- Requires no OAuth authentication
- Needs no application approval process
- Has no complex implementation requirements
- Provides immediate access through RapidAPI
Making Your First API Call
Here's how to search for LinkedIn profiles using the API:
curl --request GET \
--url https://fresh-linkedin-scraper-api.p.rapidapi.com/api/v1/search/people \
--header 'x-rapidapi-key: YOUR_RAPIDAPI_KEY' \
--header 'x-rapidapi-host: fresh-linkedin-scraper-api.p.rapidapi.com' \
--url-query 'name=John'
For JavaScript users:
const axios = require("axios");
const options = {
method: "GET",
url: "https://fresh-linkedin-scraper-api.p.rapidapi.com/api/v1/search/people",
params: {
name: "John",
page: 1,
},
headers: {
"x-rapidapi-key": "YOUR_RAPIDAPI_KEY",
"x-rapidapi-host": "fresh-linkedin-scraper-api.p.rapidapi.com",
},
};
async function searchLinkedIn() {
try {
const response = await axios.request(options);
console.log(response.data);
} catch (error) {
console.error(error);
}
}
searchLinkedIn();
Python example:
import requests
url = "https://fresh-linkedin-scraper-api.p.rapidapi.com/api/v1/search/people"
querystring = {"name":"John","page":"1"}
headers = {
"x-rapidapi-key": "YOUR_RAPIDAPI_KEY",
"x-rapidapi-host": "fresh-linkedin-scraper-api.p.rapidapi.com"
}
response = requests.request("GET", url, headers=headers, params=querystring)
print(response.json())
Understanding the Response
The API returns a JSON response with detailed information about matching profiles. Here's a sample response structure:
{
"success": true,
"message": "success",
"process_time": 884,
"data": [
{
"id": "478387397",
"urn": "ACoAAByDnMUBr75NmvcrLQrNyMolO141Kg7TD_I",
"url": "https://www.linkedin.com/in/john-heyer-685264114",
"public_identifier": "john-heyer-685264114",
"full_name": "John Heyer",
"title": "ML @ depthfirst | ex Scale / Amazon / MIT",
"location": "Boston, MA",
"is_premium": false,
"avatar": [
{
"width": 100,
"height": 100,
"url": "https://media.licdn.com/dms/image/v2/D4E03AQE8eMECAnAnsQ/profile-displayphoto-shrink_100_100/B4EZZzcHPtHQAU-/0/1745693473268?e=1752105600&v=beta&t=HqBFLGFBqPhhxdOy3onmu2SWKID67f968T3eCDeCR0Y",
"expires_at": 1752105600000
}
],
"services": []
}
// Additional profiles...
],
"cost": 1,
"page": 1,
"total": 2173900,
"has_more": true
}
Key response fields:
Field | Description |
---|---|
success | Boolean indicating if the request was successful |
cost | Cost of the request (typically 1 credit per request) |
total | Total number of results available |
has_more | Boolean indicating if there are more pages of results |
data | Array of LinkedIn profile objects |
id | LinkedIn profile ID |
urn | Unique resource identifier for the profile |
url | Direct link to the LinkedIn profile |
public_identifier | Profile username in URL |
full_name | Person's full name |
title | Professional headline |
location | Geographic location |
is_premium | Whether the profile has LinkedIn Premium |
avatar | Profile picture details |
Advanced Search Options
The API supports various search parameters to refine your results:
Parameter | Type | Description | Example |
---|---|---|---|
name | string | Search keyword for people | "John" |
page | integer | Page number for pagination (default: 1) | 1 |
geocode_location | string | Geographical code for location-based search | "103644278" |
current_company | string | Current company ID | "1441" |
past_company | string | Past company ID | "1441" |
profile_language | string | Profile language | "en" |
industry | string | Industry ID | "4" |
service_category | string | Service category ID | "602" |
Using multiple parameters together helps narrow down your search to find exactly the profiles you're looking for. For example, you could search for people named "John" who currently work at Microsoft and are located in Seattle.
Use Cases
This API enables numerous applications:
-
Talent Acquisition
- Sourcing candidates with specific skills
- Building talent pipelines for technical roles
- Finding professionals with targeted experience
-
Sales Prospecting
- Identifying potential clients by job title
- Building industry-specific lead lists
- Finding decision-makers at target companies
-
Market Research
- Analyzing professional distribution by keyword
- Tracking talent migration trends
- Conducting competitive intelligence
-
Networking
- Finding professionals with complementary skills
- Building targeted connection lists
- Identifying industry experts and thought leaders
Getting Started
To start using the LinkedIn API Search functionality:
- Sign up on RapidAPI (no credit card required)
- Subscribe to the Fresh LinkedIn Scraper API free tier
- Get your API key from the RapidAPI dashboard
- Make your first API call using the examples above
The free plan includes:
- 50 requests per month
- Rate limit: 1000 requests per hour
- Access to all endpoints including people search
Get Started with Fresh LinkedIn Scraper API Now →
FAQ
How accurate are the search results? The API returns results similar to what you would see when searching on LinkedIn directly, with matching based on keywords in profiles.
Where do I find the IDs for parameters like industry, company, or location? These IDs can be found in LinkedIn URLs when you perform searches or view profiles on the LinkedIn website. For example, company IDs appear in company profile URLs.
Can I search for connections of a specific profile? No, the API currently only supports keyword-based people search across the entire LinkedIn platform.
Does this API access private profile information? No, the API only accesses publicly available information that is visible to any LinkedIn user.
How can I get more specific results? Use multiple parameters together (name, location, company, etc.) to narrow your search results to more relevant profiles.
What happens if my search returns no results? The API will return an empty data array but still indicate success. Try broadening your search terms or removing some filters.
Is there a limit to how many profiles I can retrieve? Yes, results are paginated. Use the page parameter to navigate through all available results.