LinkedIn API Integration: Step-by-Step Guide to Using Fresh LinkedIn Scraper API on RapidAPI
Learn how to access LinkedIn profile data without OAuth using the Fresh LinkedIn Scraper API on RapidAPI. Step-by-step tutorial with code examples.
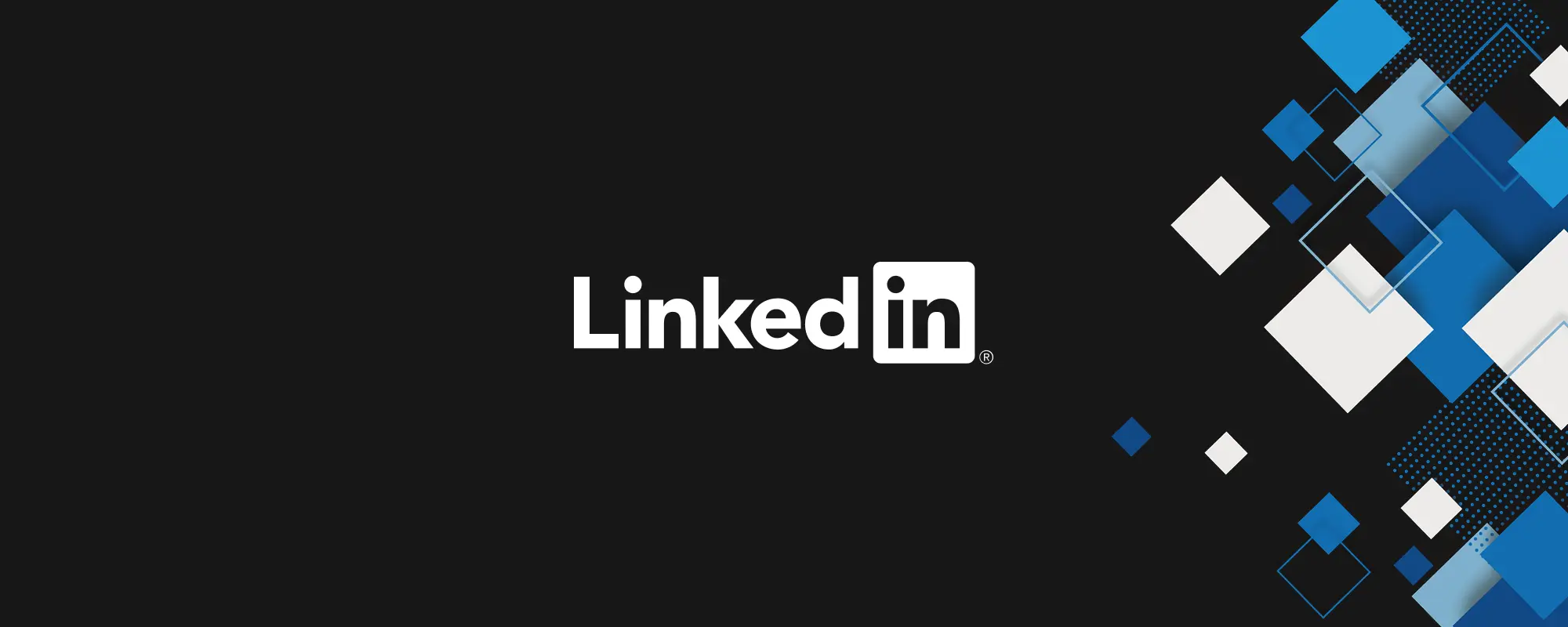
LinkedIn API Integration: Step-by-Step Guide to Using Fresh LinkedIn Scraper API on RapidAPI
Accessing LinkedIn profile data programmatically has traditionally required complex OAuth authentication and API approval processes. In this comprehensive guide, we'll explore how to integrate with the Fresh LinkedIn Scraper API on RapidAPI to easily access LinkedIn profile data without these complications.
Table of Contents
- Why Use a LinkedIn API?
- Challenges with LinkedIn's Official API
- Introducing Fresh LinkedIn Scraper API
- Step 1: Subscribe to the API
- Step 2: Choose Your Plan
- Step 3: Using the API
- Code Implementation Examples
- Understanding API Responses
- Best Practices and Rate Limiting
- Conclusion
Why Use a LinkedIn API?
LinkedIn is the world's largest professional network with over 900 million members. Programmatic access to LinkedIn data can be invaluable for:
- Recruitment: Identify and verify candidate profiles and work history
- Sales Intelligence: Research prospects and generate qualified leads
- Competitive Analysis: Monitor industry movements and key personnel changes
- Market Research: Analyze professional demographics and trends
- Personal Branding: Track profile visibility and engagement
Challenges with LinkedIn's Official API
While LinkedIn does provide official API access, it comes with significant limitations:
- Requires complex OAuth 2.0 authentication
- Limited data access compared to what's visible on public profiles
- Stringent application approval process
- Strict usage policies and volume limitations
- Requires users to authenticate through your application
These restrictions make LinkedIn's official API impractical for many business use cases where direct data access is needed.
Introducing Fresh LinkedIn Scraper API
The Fresh LinkedIn Scraper API on RapidAPI addresses these limitations by providing:
- No OAuth Required: Direct access without complex authentication
- Comprehensive Data: Access to public profile information similar to what you see on LinkedIn
- Simple Integration: RESTful API with straightforward endpoints
- Reliable Service: 98% service level with fast response times
- Flexible Plans: Options ranging from free basic access to high-volume enterprise plans
Step 1: Subscribe to the API
To get started with the Fresh LinkedIn Scraper API:
- Visit the Fresh LinkedIn Scraper API on RapidAPI
- Click on the "Subscribe to Test" button in the top right corner of the page
- You'll need to create a RapidAPI account if you don't already have one
- Once logged in, you'll be presented with available subscription plans
Step 2: Choose Your Plan
The API offers several pricing tiers to match different usage needs:
-
Review the available plans on the pricing page:
- Basic Plan: Free access with 50 requests/month and limited rate (1000 requests/hour)
- Pro Plan: $50/month for 20,000 requests with increased rate limits (20 requests/minute)
- Ultra Plan: $200/month for 100,000 requests with higher throughput (120 requests/minute) - Recommended for business applications
- Mega Plan: $500/month for 500,000 requests with maximum performance (300 requests/minute)
-
Select the plan that best fits your requirements by clicking the corresponding "Choose This Plan" button
- For testing purposes, you can start with the Basic plan by clicking "Start Free Plan"
- For production use, the Ultra plan offers the best balance of volume and performance
-
Complete the subscription process by following the payment instructions (if selecting a paid plan)
Step 3: Using the API
After subscribing, you'll have access to your API key and can start making requests:
Exploring Available Endpoints
The API provides several endpoints for different LinkedIn data needs:
- Get User Profile: Retrieve comprehensive profile data for a specific user
- Search People: Find LinkedIn users based on various criteria
- Get User Posts: Retrieve a user's activity and posts
- Get User Comments: Access comments made by a specific user
- Get User Reactions: See a user's engagement with content
- Get User Images: Retrieve profile and background images
- Get User Recommendations: Access recommendations received or given
Making Your First API Call with the Search People Endpoint
Let's walk through how to use the "Search People" endpoint:
-
Navigate to the "Search People" endpoint in the RapidAPI dashboard by clicking on it in the left sidebar
-
You'll see the endpoint details with parameters, code snippets, and example responses
-
The endpoint accepts these parameters:
name
(optional): Search keyword for people (e.g., "john")page
(optional): Page number for pagination (default: 1)geocode_location
(optional): Geographical code for location-based search (e.g., "103644278" for United States)current_company
(optional): Current company ID to filter resultspast_company
(optional): Past company ID to filter results
-
To test the endpoint directly from the dashboard:
- Enter parameter values (e.g., name: "john", page: "1")
- Click the "Test Endpoint" button
- Review the response to see the LinkedIn profile data returned
Code Implementation Examples
Here's how to implement the API in various programming languages:
Node.js Example
const axios = require("axios");
const options = {
method: "GET",
url: "https://fresh-linkedin-scraper-api.p.rapidapi.com/api/v1/search/people",
params: {
name: "john",
page: "1",
},
headers: {
"x-rapidapi-key": "YOUR_API_KEY",
"x-rapidapi-host": "fresh-linkedin-scraper-api.p.rapidapi.com",
},
};
async function fetchData() {
try {
const response = await axios.request(options);
console.log(response.data);
} catch (error) {
console.error(error);
}
}
fetchData();
Python Example
import requests
url = "https://fresh-linkedin-scraper-api.p.rapidapi.com/api/v1/search/people"
querystring = {"name":"john","page":"1"}
headers = {
"x-rapidapi-key": "YOUR_API_KEY",
"x-rapidapi-host": "fresh-linkedin-scraper-api.p.rapidapi.com"
}
response = requests.request("GET", url, headers=headers, params=querystring)
print(response.text)
Understanding API Responses
The API returns comprehensive JSON data. Here's what a typical response structure looks like:
{
"status": "success",
"data": {
"profiles": [
{
"profile_id": "john-doe-123456",
"full_name": "John Doe",
"headline": "Software Engineer at Example Corp",
"location": {
"country": "United States",
"city": "San Francisco"
},
"profile_url": "https://www.linkedin.com/in/john-doe-123456/",
"profile_image": "https://media.licdn.com/dms/image/...",
"current_company": "Example Corp",
"experience": [
{
"company": "Example Corp",
"title": "Software Engineer",
"start_date": "2021-01"
}
]
}
// Additional profiles...
],
"pagination": {
"current_page": 1,
"total_pages": 10
}
}
}
Best Practices and Rate Limiting
When working with the API, follow these best practices:
Implement Proper Error Handling
try {
const response = await axios.request(options);
handleSuccessfulResponse(response.data);
} catch (error) {
if (error.response) {
// The server responded with a status code outside the 2xx range
console.error(
`Error ${error.response.status}: ${error.response.data.message}`
);
if (error.response.status === 429) {
// Handle rate limit exceeded
const resetTime = error.response.headers["x-ratelimit-reset"];
console.log(
`Rate limit exceeded. Try again after ${new Date(resetTime * 1000)}`
);
}
} else if (error.request) {
// The request was made but no response was received
console.error("No response received from API");
} else {
// Something happened in setting up the request
console.error("Error setting up request:", error.message);
}
}
Respect Rate Limits
Monitor your usage through the RapidAPI dashboard and implement backoff strategies:
// Exponential backoff function
const sleep = (ms) => new Promise((resolve) => setTimeout(resolve, ms));
async function fetchWithRetry(options, maxRetries = 3) {
let retries = 0;
while (retries < maxRetries) {
try {
return await axios.request(options);
} catch (error) {
if (error.response && error.response.status === 429) {
// Rate limit exceeded - wait and retry
const backoffTime = Math.pow(2, retries) * 1000;
console.log(`Rate limited. Retrying in ${backoffTime}ms...`);
await sleep(backoffTime);
retries++;
} else {
throw error;
}
}
}
throw new Error("Maximum retries exceeded");
}
Cache Responses
Implement a caching mechanism to reduce the number of API calls:
const NodeCache = require("node-cache");
const cache = new NodeCache({ stdTTL: 3600 }); // Cache for 1 hour
async function getLinkedInProfile(profileId) {
// Check if data exists in cache
const cachedData = cache.get(profileId);
if (cachedData) {
console.log("Returning cached data");
return cachedData;
}
// If not in cache, fetch from API
const options = {
method: "GET",
url: "https://fresh-linkedin-scraper-api.p.rapidapi.com/api/v1/user/profile",
params: { username: profileId },
headers: {
"x-rapidapi-key": "YOUR_API_KEY",
"x-rapidapi-host": "fresh-linkedin-scraper-api.p.rapidapi.com",
},
};
const response = await axios.request(options);
// Store in cache
cache.set(profileId, response.data);
return response.data;
}
Conclusion
The Fresh LinkedIn Scraper API provides a powerful and straightforward way to access LinkedIn profile data without the complexity of OAuth authentication. By following the steps outlined in this guide, you can quickly integrate LinkedIn data into your applications for recruitment, sales intelligence, market research, and more.
Remember to respect LinkedIn's terms of service and use the obtained data responsibly. The API provides access to publicly available information, but it's essential to handle user data ethically and in compliance with privacy regulations.
Ready to get started? Subscribe to the Fresh LinkedIn Scraper API on RapidAPI and begin integrating LinkedIn data into your applications today.