How to Get LinkedIn Posts Using API?
Learn how to retrieve LinkedIn user profiles and posts data using the LinkedIn Scraper API with simple Node.js examples.
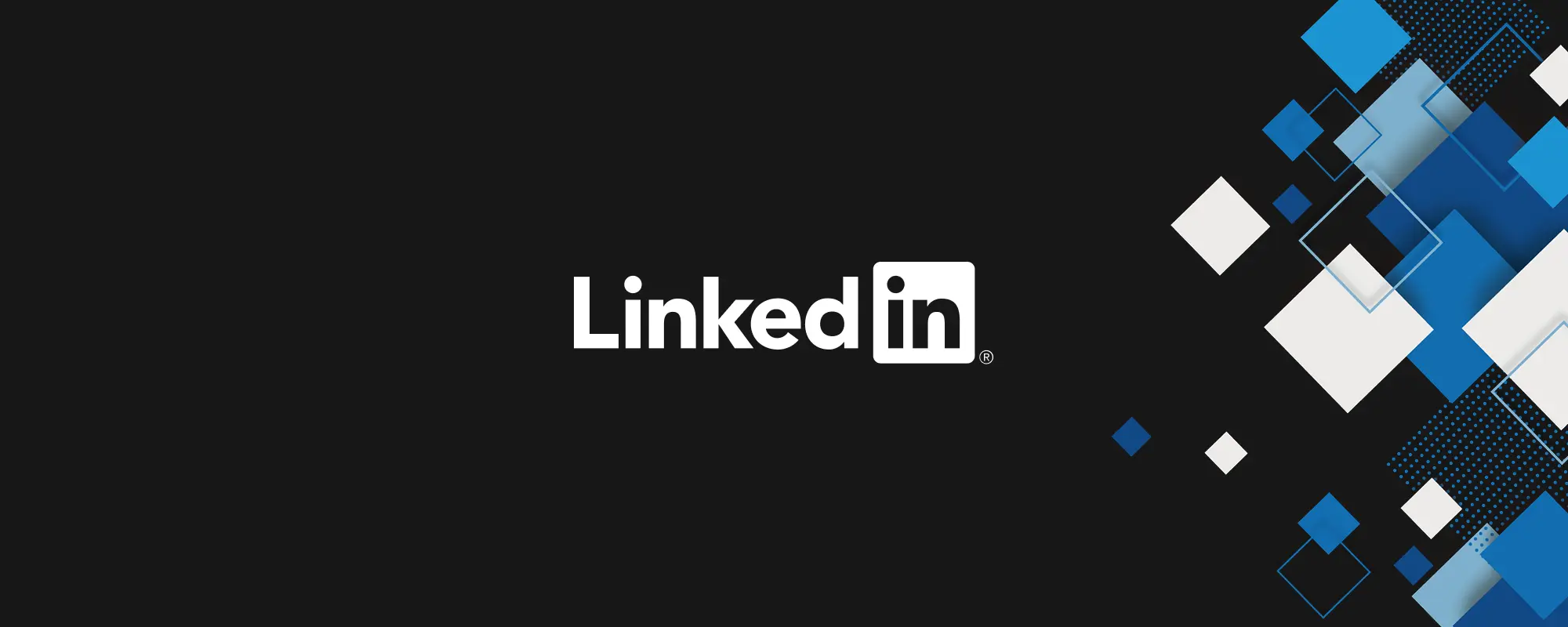
How to Get LinkedIn Posts Using API?
Accessing LinkedIn data programmatically can be valuable for various applications, from social media monitoring to market research. In this guide, we'll explore how to retrieve LinkedIn posts using the Fresh LinkedIn Scraper API available on RapidAPI.
Prerequisites
Before getting started, you'll need:
- A RapidAPI account
- Access to the Fresh LinkedIn Scraper API
- Node.js installed on your machine
- Basic understanding of JavaScript/Node.js
Step 1: Retrieve a User's LinkedIn Profile Information
To get a user's posts, you first need their unique identifier known as "URN" (Uniform Resource Name). This can be obtained by fetching the user's profile using their LinkedIn username.
Here's how to get a user's profile information:
const axios = require("axios");
const options = {
method: "GET",
url: "https://fresh-linkedin-scraper-api.p.rapidapi.com/api/v1/user/profile",
params: {
username: "williamhgates", // Bill Gates' LinkedIn username as an example
},
headers: {
"x-rapidapi-key": "YOUR_RAPIDAPI_KEY",
"x-rapidapi-host": "fresh-linkedin-scraper-api.p.rapidapi.com",
},
};
async function fetchUserProfile() {
try {
const response = await axios.request(options);
console.log(response.data);
// Extract and save the URN for later use
const urn = response.data.data.urn;
console.log(`User URN: ${urn}`);
return urn;
} catch (error) {
console.error("Error fetching user profile:", error);
}
}
fetchUserProfile();
Example Response
Here's an example of the response you'll receive when fetching Bill Gates' profile:
{
"success": true,
"message": "success",
"process_time": 468,
"cost": 1,
"data": {
"id": "251749025",
"urn": "ACoAAA8BYqEBCGLg_vT_ca6mMEqkpp9nVffJ3hc",
"public_identifier": "williamhgates",
"first_name": "Bill",
"last_name": "Gates",
"full_name": "Bill Gates",
"headline": "Chair, Gates Foundation and Founder, Breakthrough Energy",
"is_premium": true,
"is_open_to_work": false,
"is_hiring": false,
"is_memorialized": false,
"is_influencer": true,
"is_top_voice": true,
"is_creator": true,
"birth": {
"day": null,
"month": null,
"year": null
},
"pronoun": null,
"created": 1367521780840,
"created_date": "2013-05-02T19:09:40.840Z",
"location": {
"country": "United States",
"country_code": "US",
"city": "Seattle, Washington, United States",
"postal_code": null
},
"avatar": [
{
"width": 200,
"height": 200,
"url": "https://media.licdn.com/dms/image/v2/D5603AQF-RYZP55jmXA/profile-displayphoto-shrink_200_200/B56ZRi8g.aGsAY-/0/1736826818802?e=1749081600&v=beta&t=8hZGHekNOCdOgVucnR22cbwafY_kXLCmIDVl3DGkfSc",
"expires_at": 1749081600000
},
{
"width": 400,
"height": 400,
"url": "https://media.licdn.com/dms/image/v2/D5603AQF-RYZP55jmXA/profile-displayphoto-shrink_400_400/B56ZRi8g.aGsAg-/0/1736826818802?e=1749081600&v=beta&t=COIAnqFqPM_6v8kTUnIdUV0ymqE-iv8ehHl_Kr0h5SQ",
"expires_at": 1749081600000
},
{
"width": 100,
"height": 100,
"url": "https://media.licdn.com/dms/image/v2/D5603AQF-RYZP55jmXA/profile-displayphoto-shrink_100_100/B56ZRi8g.aGsAU-/0/1736826818802?e=1749081600&v=beta&t=hd4wzNKgmLsnVnM3UrvKucxHcLYV2m7Az4aB-AFHrq8",
"expires_at": 1749081600000
},
{
"width": 800,
"height": 800,
"url": "https://media.licdn.com/dms/image/v2/D5603AQF-RYZP55jmXA/profile-displayphoto-shrink_800_800/B56ZRi8g.aGsAc-/0/1736826818808?e=1749081600&v=beta&t=NbWZseAYCZLDFblO75TLDt0LEGF5kjLB3mOkve_XNrg",
"expires_at": 1749081600000
}
],
"cover": [
{
"width": 800,
"height": 200,
"url": "https://media.licdn.com/dms/image/v2/D5616AQEyKmz7jPZung/profile-displaybackgroundimage-shrink_200_800/profile-displaybackgroundimage-shrink_200_800/0/1737141827999?e=1749081600&v=beta&t=6vV54NhapnL2PqnzQH6mgBJzcQmzwY-ekqwcu1zkORQ",
"expires_at": 1749081600000
},
{
"width": 1400,
"height": 350,
"url": "https://media.licdn.com/dms/image/v2/D5616AQEyKmz7jPZung/profile-displaybackgroundimage-shrink_350_1400/profile-displaybackgroundimage-shrink_350_1400/0/1737141827999?e=1749081600&v=beta&t=OkbwMcdTgO3hoCPxhkp2cA18p6jo7y8r5EcvyAlIZLQ",
"expires_at": 1749081600000
}
],
"associated_hashtag": [
"#books",
"#healthcare",
"#innovation",
"#climatechange",
"#sustainability"
],
"website": {},
"supported_locales": [
{
"country": "US",
"language": "en"
}
],
"primary_locale": {
"country": "US",
"language": "en"
}
}
}
As you can see, the response contains comprehensive information about the user's profile. The most important value for our purpose is the urn
field (ACoAAA8BYqEBCGLg_vT_ca6mMEqkpp9nVffJ3hc
in this case), which we'll need to fetch the user's posts.
Step 2: Fetch the User's LinkedIn Posts
Once you have the user's URN, you can retrieve their posts using another endpoint:
const axios = require("axios");
async function fetchUserPosts(urn) {
const options = {
method: "GET",
url: "https://fresh-linkedin-scraper-api.p.rapidapi.com/api/v1/user/posts",
params: {
urn: urn, // Use the URN obtained from the profile request
page: "1", // Pagination parameter - start with page 1
},
headers: {
"x-rapidapi-key": "YOUR_RAPIDAPI_KEY",
"x-rapidapi-host": "fresh-linkedin-scraper-api.p.rapidapi.com",
},
};
try {
const response = await axios.request(options);
console.log(response.data);
return response.data;
} catch (error) {
console.error("Error fetching user posts:", error);
}
}
// Example of combining both functions
async function getLinkedInPosts() {
// First get the user profile to extract the URN
const urn = await fetchUserProfile();
// Then use the URN to fetch posts
if (urn) {
const posts = await fetchUserPosts(urn);
console.log("Posts retrieved successfully!");
}
}
getLinkedInPosts();
Example Posts Response
Here's an example of the response you'll receive when fetching a user's posts:
{
"success": true,
"message": "success",
"process_time": 831,
"cost": 1,
"page": 1,
"pagination_token": "dXJuOmxpOmFjdGl2aXR5OjcyNDk0MzI2MDY2MjA5NzUxMDQtMTcyODM5OTQyMTMzNQ==",
"data": [
{
"id": "7310539768939847681",
"text": "It takes me exactly 21 days to prepare for coding interviews.\n\nI have a simple system: 100 problems in 3 weeks.\n\nFive problems every day. No more, no less.\n\nIt might sound like a lot, but there's a method to this madness.\n\nI stick to Python for all my interview prep. No complex syntax. No extra boilerplate code. Just pure problem-solving in its simplest form.\n\nMost of my practice comes from popular interview questions. After years in tech, these problems start to feel familiar. Different companies often ask variations of the same questions.\n\nBut here's what really makes the difference.\n\nIn my 7 years as a software engineer, I've failed more than 100 interviews. Those 100 failures have taught me more than 1000 practice problems could.\n\nI don't need mock interviews anymore. My real interview experiences have been the best teachers.\n\nExperience is the best interview prep you can't buy.\n\nBtw, if you want to become a better engineer in 5 minutes per week, try my email newsletter: https://lnkd.in/gPjuaY9w\n\n#leetcode #codinginterviews",
"content": {
"images": [
{
"image": [
{
"width": 160,
"height": 213,
"url": "https://media.licdn.com/dms/image/v2/D5622AQFKXBgPKZHOjA/feedshare-shrink_160/B56ZXRBLa3GQAc-/0/1742968502590?e=1746057600&v=beta&t=L6y-8YpA28-ZXEUicUZnt5Dw3Yfts0BGZi9J_ZL9P24",
"expires_at": 1746057600000
}
]
}
],
"video": null,
"document": null,
"article": null,
"celebration": null,
"poll": null,
"event": null
},
"activity": {
"num_likes": 10688,
"num_comments": 215,
"num_shares": 53,
"reaction_counts": [
{
"count": 10008,
"type": "LIKE"
},
{
"count": 305,
"type": "EMPATHY"
},
{
"count": 192,
"type": "INTEREST"
},
{
"count": 126,
"type": "PRAISE"
},
{
"count": 42,
"type": "APPRECIATION"
},
{
"count": 15,
"type": "ENTERTAINMENT"
}
]
},
"author": {
"id": "279808191",
"urn": "ACoAABCtiL8B26nfi3Nbpo_AM8ngg4LeClT1Wh8",
"url": "https://www.linkedin.com/in/gabag26",
"public_identifier": "gabag26",
"first_name": "Sahil",
"last_name": "Gaba",
"full_name": "Sahil Gaba",
"description": "Software Engineer at Google | Amazon",
"avatar": [
{
"width": 100,
"height": 100,
"url": "https://media.licdn.com/dms/image/v2/C5603AQGo7vmLHtq8Lw/profile-displayphoto-shrink_100_100/profile-displayphoto-shrink_100_100/0/1626677360609?e=1749081600&v=beta&t=wLd5asaLBgoqm-OmAxuW7X4ONzhY3bcFmm1vY7eNkyw",
"expires_at": 1749081600000
}
],
"account_type": "user"
},
"created_at": "2025-03-27T03:13:06.973Z",
"url": "https://www.linkedin.com/feed/update/urn:li:activity:7310539768939847681",
"share_urn": "urn:li:share:7310539765131395072"
},
{
"id": "7308341413136502784",
"text": "This is the resume that got me into Google.\n\nHere are a few things to keep in mind when writing your resume:\n\nMost people will not read your full resume. Make the first few sentences count.\n\nWhen talking about your projects, answer 3 questions: What did you do, How did you do it and What was the impact?\n\nFor a quick overview of your profile, write a separate 'Skills' section.\n\nUse simple easy to understand language. Don't use words like 'spearheaded' or 'championed'. I have made this mistake in my own resume.\n\nYou can write an 'Interests' section if you want to help interviewers find ice breakers.\n\nKeep it short and sweet. One page is enough.\n\nIf I could tell you only one thing about Resumes, it would be this:\n\nResumes are not just written. Resumes are built over time. So, take your time to build a resume that will get you your 'big break'. Good luck!\n\nBtw, if you want to become a better engineer in 5 minutes per week, try my email newsletter: https://lnkd.in/gPjuaY9w\n\n#LifeAtGoogle #Resume #SoftwareEngineering",
"created_at": "2025-03-24T03:13:06.973Z",
"url": "https://www.linkedin.com/feed/update/urn:li:activity:7308341413136502784",
"share_urn": "urn:li:share:7308341411253207041"
}
]
}
As you can see, the response contains an array of post objects with detailed information about each post:
- Post content: Full text of the post in the
text
field - Media content: Images, videos, or other media attached to the post
- Engagement metrics: Number of likes, comments, shares, and reaction types
- Author information: Details about the post author
- Post metadata: Creation date, URLs, and unique identifiers
The response also includes pagination information like page
and pagination_token
, which you can use to fetch additional pages of posts.
Understanding the Response
When you fetch a user's profile, you'll receive detailed information including:
- User ID and URN
- Public identifier (username)
- Full name and headline
- Account status (premium, influencer, etc.)
- Profile pictures and cover images
- Location information
- Associated hashtags
The post retrieval endpoint returns:
- Post content
- Images or attachments
- Engagement metrics (likes, comments, etc.)
- Posting date and time
- Post type and visibility
Handling Pagination
If a user has many posts, they'll be spread across multiple pages. You can iterate through pages by updating the page
parameter:
async function fetchAllUserPosts(urn) {
let allPosts = [];
let currentPage = 1;
let hasMorePages = true;
while (hasMorePages) {
const options = {
method: "GET",
url: "https://fresh-linkedin-scraper-api.p.rapidapi.com/api/v1/user/posts",
params: {
urn: urn,
page: currentPage.toString(),
},
headers: {
"x-rapidapi-key": "YOUR_RAPIDAPI_KEY",
"x-rapidapi-host": "fresh-linkedin-scraper-api.p.rapidapi.com",
},
};
try {
const response = await axios.request(options);
const posts = response.data.data.posts;
if (posts && posts.length > 0) {
allPosts = [...allPosts, ...posts];
currentPage++;
} else {
hasMorePages = false;
}
} catch (error) {
console.error(`Error fetching page ${currentPage}:`, error);
hasMorePages = false;
}
}
return allPosts;
}
Best Practices
- Rate Limiting: Be mindful of the API's rate limits to avoid getting your requests blocked.
- Error Handling: Implement robust error handling to gracefully manage API failures.
- Secure API Keys: Never expose your RapidAPI key in client-side code.
- Caching: Consider caching responses to minimize API calls and reduce costs.
Conclusion
The LinkedIn Scraper API provides a straightforward way to programmatically access LinkedIn user profiles and posts data. By following this guide, you can integrate LinkedIn data into your applications for analysis, monitoring, or other purposes.
Remember to review the API provider's terms of service and ensure your usage complies with LinkedIn's platform policies.